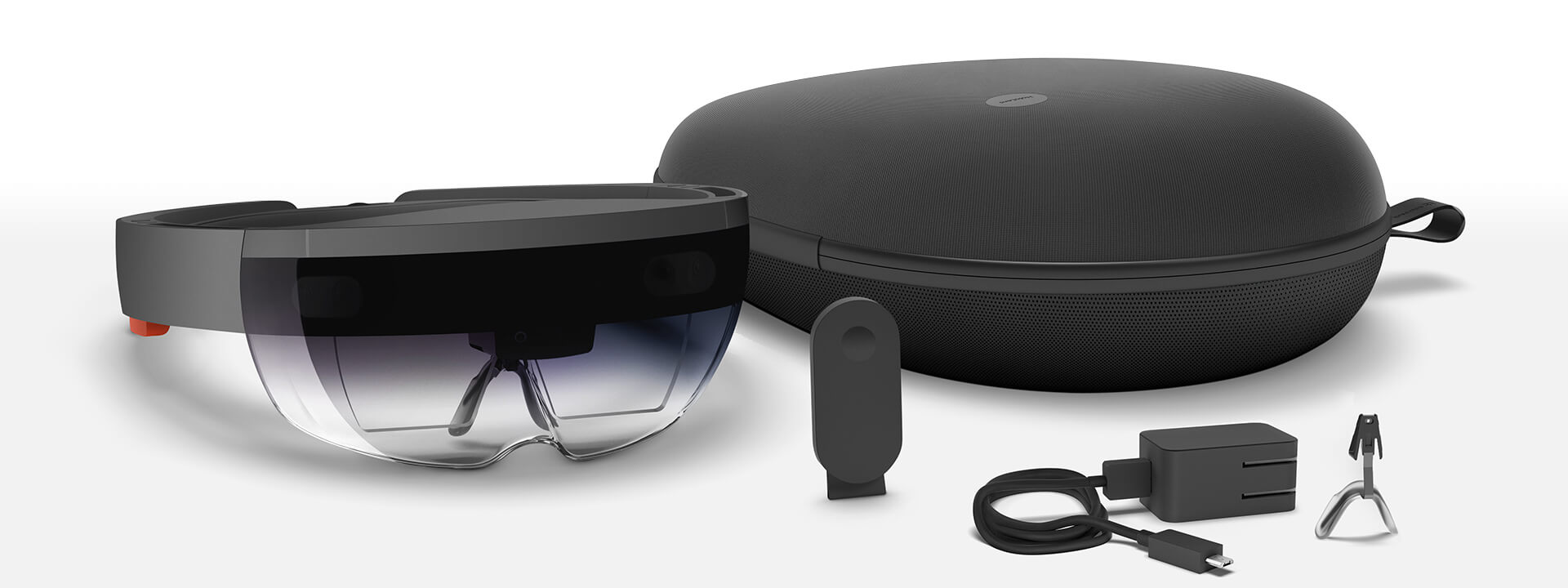
Augmented reality is increasingly being used as an assistive tool in training and education. During these trials, researchers have found potential positives in increases in interaction. Despite this, there has also been evidence showing augmented reality can negatively affect our attention. Seeing that augmented reality can affect our perception, it is important to investigate how the medium affects our perception of distractions, as distraction has been shown in educational settings to be a barrier for learning. However, these effects on our attention can be leveraged to the benefit of the user. In this study, we assess if the platform being used in an environment with or without distractions affected the learning retention of participants viewing a virtual lecture. While there is no statistical significance between platforms, distractions, and the scores obtained by users on the quiz, the feedback from users and the number of reported distractions provides evidence for the concept of inattentional blindness and deafness playing a pivotal role in how users overcome distractions and even how they focus on information at a certain point in time.
Requirements to perform the study:
- A VR Headset (in our case, Microsoft Holo Lens)
- Laptop
- A Closed Environment
- Internet Connection
- Snacks and Refreshers for participants!
Participants' Prerequisite:
- No prior knowledge of the Lecture Topic
- Should posses English Language Skills
Overview of the Study
We started out by selecting a Historical Audio Lecture based on Ancient Rome. We used this audio file to create 2 different lecture formats:
- 2D Lecture (to be played on a Laptop screen)
- 2D Lecture(to be played on a VR headset)
The participants were asked to listen to either forms of the video lectures. At the end of the lecture, they were asked to fill out a MCQ based Questionnaire. These participants were divided into 4 groups : screen with distractions, screen without distractions, HoloLens with distractions, and HoloLens without distractions. The Distractions were introduced intentionally by the Study team specific times so as to ensure that they do not hinder the student from actually being able to hear the answers to the specific questions(around a 10 sec timestamp of the answer). We limited our distractions to mobile ringtones, people walking in the room and people coming in through the door. We had a total of 40 participants in all with 2 different platform groups. The first group with the Hololens and second with the Laptop Screen.
Preprocessing the VR Environment:
Initial Prototype of our Virtual Professor:
Final view from Hololens:
Result:
Addressing questions answered correctly out of nine, the mean
value for the Monitor Control group was 6. Among the group, he
standard deviation was 2 and the variance was 4. The mean value
for the Monitor Distraction group was 5.5. Among the group, the
standard deviation was 2.37 and the variance was 5.61. The mean
value for the HoloLens Control group was 6.4. Among the group,
the standard deviation was 2.27 and the variance was 5.16. The
mean value for the HoloLens Distraction group was 5.7. Among the
group, the standard deviation was 1.49 and the variance was 2.2.

The goal of this experiment was to test the benefits of augmented
reality in overcoming environment distractions. Since classroom
distractions can occur frequently in real-world scenarios, a case can
be made to suggest that using augmented reality to increase
inattentional blindness and deafness can provide great benefits for
instructors’ control over a classroom setting, as well as students’
retention of knowledge.
From this experiment, we gathered data regarding distractions
on each platform. After reviewing and analyzing the data, and
seeing no statistical differences surrounding platform and
distraction on quiz scores, we saw evidence of inattentional
blindness and deafness based on reported distractions. Our research
question attempts to provide an analysis of which platform would
help students to recover more efficiently or more readily from distractions. The data we collected underscores the positives of
inattention and provides insight into the benefits of unconventional
tools like the HoloLens and their utility in a classroom setting.
After witnessing the experiences in these environments and
reviewing feedback provided about each platform’s lecture, we
believe certain aspects of augmented reality can be leveraged in a
learning environment to the benefit of the user. Some attributes of
the HoloLens can be a hindrance to the user, such as comfortability
and eye strain. However, interaction capabilities of the HoloLens
and similar platforms offer a wide range for user engagement in the
environment, enticing increased attentiveness on the subject matter
among learners using these tools.
Although the data presented in this paper is preliminary, it
stands to reason that with more participants, we can start to see
increased trends among them. Along with this, we could see more
significant differences between performance of users when using
the HoloLens and being distracted or not. With more participants
and test questions that have been verified for their difficulty,
actionable data can be acquired to argue for the implementation for
these tools to be incorporated into different modalities in various
learning environments.
To view the entire paper, please
click here.
Finally I would like to express my gratitudes to my teammates : Emma Drobina, Jacob Stuart and Kieth McNamara Jr.